Discover the power of Python comments in your coding journey! Python, a popular and versatile language, utilizes comments in code to increase readability and enhance collaboration. Particularly when crafting games or working on larger projects, the strategic use of comments can be the guiding light for both your present self and future collaborators.
Table of contents
What is the Concept of Comments in Python?
In Python, a comment is a piece of text in your code that isn’t executed when the program runs. These text snippets start with a # symbol, and are largely used for explaining or clarifying the code.
At first glance, writing explanations for code may seem inconsequential. However, as your projects grow in complexity, these written hints prevent you from getting lost in your own code. Furthermore, when you’re working in a team or contributing to open-source projects, comments serve as a communication medium to fellow developers.
Why Should You Learn to Use Python Comments?
Learning to use comments efficiently can make the difference between a project that’s a joy to develop and maintain, and one that’s a headache. Moreover, it’s an expectation in the professional world, as clear comments would:
- Help you understand your code after a long pause
- Assist others to understand and contribute to your project faster
- Document functionality and rationale behind code snippets
Understanding the power of Python comments is a key step towards better collaborative coding and self-organization. Now, let’s dive deeper into the topic with practical examples.
Single Line Comments in Python
Single line comments in Python start with the hash symbol (#). Here’s a simple example:
# This is a single line comment print("Hello, Zenva!")
Here, Python will ignore the comment line and only execute the print statement.
Inline Comments in Python
Inline comments are placed on the same line as the code statement. They’re used to clarify specific lines of code. Let’s demonstrate with an example:
print("Hello, Zenva!") # This will print "Hello, Zenva!" to the console
Multiple Line Comments in Python
When documenting multiple lines of code, you’d use multiple line comments. Python doesn’t have a built-in syntax for multi-line comments, but we can leverage the tripe quotes (”’ or “””) multi-line strings for this purpose:
''' This comment spans multiple lines. Python will ignore these lines ''' print("Hello, Zenva!")
Comments for Function Definition
Comments are also vital when defining functions. They help describe the role of the function, the expected input parameters, and the return value. This is known as the function’s docstring:
def add(x, y): """ This function adds two numbers. Input: Two numeric values Returns: The sum of the input values """ return x+y
In the above code, the text between the triple quotes is the function’s docstring, providing valuable information about the function.
Docstrings for Class Definition
Just like functions, classes in Python often come with docstrings to describe their purpose and functionality. Here’s an example:
class ZenvaAcademy: """ This class represents Zenva Academy. Zenva Academy offers courses in several coding disciplines. """ pass
In this instance, the docstring gives some context about what the ZenvaAcademy class is for.
Conditional Comments
Sometimes, we write comments in a way that not only humans, but also Python interpreters can read. This becomes useful when you need different code to execute depending on the Python version or other conditions. Take the following into account:
import sys if sys.version_info[0] < 3: # This block will run only if Python version is less than 3 print("You are using Python 2.x") else: # This block will run in Python 3.x print("You are using Python 3.x")
This code uses inline comments to clarify what each section is doing.
Commenting Out Code
Another common use for comments in Python is to “turn off” parts of the code temporarily, which is particularly useful when debugging your code:
print("This will print") #print("This will not print")
In this example, the second print statement will not execute because it is commented out.
Understanding Comments: TODO, FIXME, and HACK
In the professional programming environment, you’ll often come across comments carrying TODO, FIXME, or HACK:
# TODO: refactor this code to remove redundancy # FIXME: this function fails when input is a negative number # HACK: this is a quick and dirty solution, redo later
These comments are special markers indicating tasks to do (TODO), things to fix (FIXME), or areas where the solution may not be ideal and requires attention (HACK).
We hope this gives you a comprehensive idea about the power and importance of comments in Python. Whether you’re coding a simple script or a complex game, effective use of comments can streamline your development process and make collaboration a breeze.
How to Keep Learning
Now you understand the significance of Python comments and how to use them adequately. The next step is to continue learning and practising. Incorporating comments into your daily coding habits is the key to mastering this important aspect of writing clear, comprehensive code.
To fully take advantage of Python’s power and versatility, check out our Python Mini-Degree. This comprehensive collection of Python courses doesn’t just touch upon the basics of coding. It delves deep into crucial topics such as algorithms, object-oriented programming, game development, app creation, and more! By creating your own games, algorithms, and real-world apps, you assimilate these concepts more effectively.
Irrespective of whether you’re a beginner or an experienced programmer, the Python Mini-Degree is suitable for you. Our courses are flexible, allowing you to learn at your own pace and skip ahead to lessons most relevant to your needs. The courses, designed by experienced coders and gamers, include interactive lessons, quizzes, and coding challenges that enhance your learning experience.
For a broader exploration of Python, don’t hesitate to browse our extensive collection of Python courses.
Conclusion
Mastering Python comments enhances your ability to write easy-to-understand code that’s well-documented, collaborative-friendly, and simple to maintain. This lays the groundwork for more efficient coding, whether you’re creating games, building apps, or contributing to open-source projects. So why not dive in and start swimming deeper into the Python pool?
Remember that learning to code is a journey, and we at Zenva are here to guide you every step of the way. Don’t miss the opportunity to explore our Python Mini-Degree and take your Python skills to the next level. Together, let’s make your coding dreams a reality!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
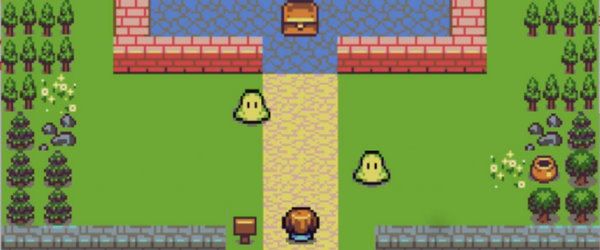
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.